July 25, 2006
SuperGraphics supports gradient & pattern fills. Currently, only linear gradients have been implemented in the SVG and CoreGraphics implementation.
Basic Usage
Gradients are defined by creating a SuperGraphicsGradient object and inserting “Gradient Stops” — key points at which define the color interpolation. This is done by calling the SuperGraphicsGradient.InsertStop method, which takes the following parameters:
sOffset as Double, sColor as TinrocketColor, sMidpoint as Double = 0.5
So, to explain the parameters:
- sOffset is the location along the gradient 0.0 to 1.0.
- sColor is a TinrocketColor. A note about color: This class supports colors in RGBA format. TinrocketColorCMYK supports CMYKA color definitions. Both TinrocketColor and TinrocketCMYK objects can be used interchangeably within SuperGraphics — each class is able to convert itself to an RGB or CMYK color model as needed.
- sMidpoint is an optional parameter between 0.0 and 1.0 which weighs the gradient closer to either stop. A default value of 0.5 means that the blend will be an equally weighted, linear blend.
Example
Below is sample code that creates a SuperGraphicsGradient object and defines five gradient stops. Gradient stops may be inserted in any order — the class will arrange them properly based on the sOffset parameter.
dim sg as SuperGraphicsGradient sg = new SuperGraphicsGradient //Inserting stops out of order to test insertion code sg.InsertStop(0.0,&cFFFFFF,.7) //First sg.InsertStop(0.25,&cFF0000,.7) //Second sg.InsertStop(1.0,&c000000) //Fifth sg.InsertStop(0.5,&c0000FF,.1) //Third sg.InsertStop(0.75,&c00FF00) //Fourth me.superGraphicsObject.FillGradient = sg
SuperGraphics will use this gradient object to fill all subsequent paths until the SuperGraphics.FillGradient property is set to nil.
Other Features
There are a few other things involved with using the SuperGraphicsGradient class:
- SuperGraphicsGradient.Vector is a TinrocketVector2D object that will define the direction of the gradient.
- By default, the gradient will scale to the bounding box of the shape that it fills. This can be overridden by setting SuperGraphicsGradient.ScaleToShapeBoundingBox to FALSE and setting the SuperGraphicsGradient.Origin and SuperGraphicsGradient.Vector properties to position the gradient.
- SuperGraphicsGradient.Pad can be set to one of three constants which tell SuperGraphics what to do when it has to fill areas before and after the normal gradient range of 0.0 to 1.0. The options are: kPad_None, kPad_Reflect, & kPad_Repeat
- For SuperGraphics implementations that do not support the concept of gradient midpoints, such as SVG, SuperGraphics can flatten non-linear midpoint blends into series of linear blends.
- SuperGraphics interpolates individual color components. Hue interpolation is a possible feature in the future.
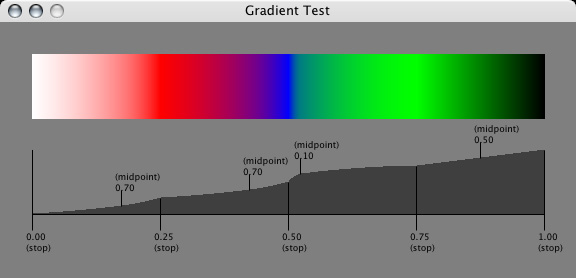
This is the gradient produced by the code above when drawn inside a rectangle. The curve below is the profile of the gradient, showing the gradient stop and midpoint positions, as well as the curves produced by various midpoint settings. The midpoint with a value of 0.5 between gradient stops 0.75 and 1.0 is a linear blend.